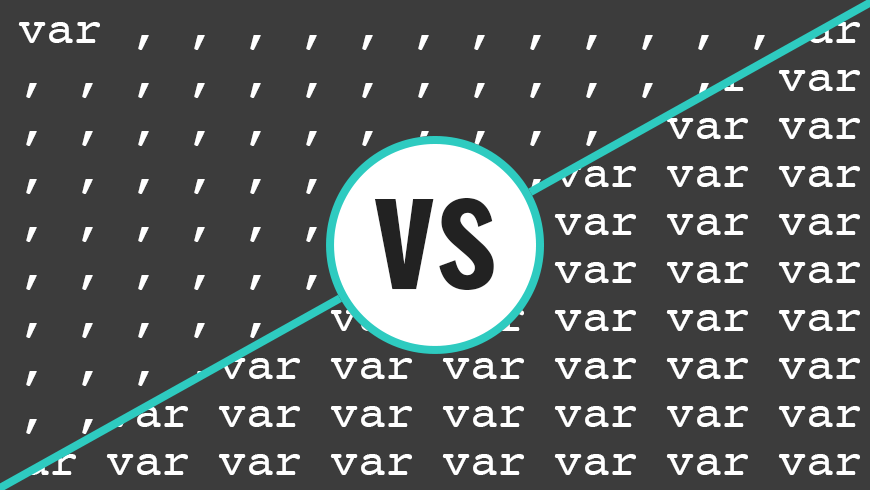
In programming, there’s always little stylistic differences that differentiate one coder from another. Often these differences create opposing camps where the members of each are quite passionate about how their style is the one true way to code. Tabs vs spaces; OOP vs functional; cuddling braces vs on a new line. Here we’ll be discussing how we define our variables in JavaScript.
There are two distinct camps:
All variables declared with a single var
statement.
This method can actually be broken down further into a more extreme and less extreme version.
var jedis = ['luke', 'obi-wan', 'yoda'], sith = ['vader', 'maul', 'sidious'], force = 'with you', x, y, width, height;
var jedis = ['luke', 'obi-wan', 'yoda'], sith = ['vader', 'maul', 'sidious'], force = 'with you', x, y, width, height;
vs
Each variable declared separately.
var jedis = ['luke', 'obi-wan', 'yoda']; var sith = ['vader', 'maul', 'sidious']; var force = 'with you'; var x; var y; var width; var height;
Syntactically, these are all identical, but there are pros and cons to each. However, I aim to convince you that the pros of one vastly outweigh the other. First, let’s address each of the possible arguments someone might use to convince you to use one method vs the other.
Argument 1: Single var
statements look better
Although this is a totally subjective opinion, I would have to agree. I prefer the look of having a single var
statement. It just looks more elegant when you type the bare minimum needed to make your code valid. It especially looks best when variables are tightly coupled together in terms of their use, such as x/y
, width/height
, etc…
However, this is only ever true if you’re using 4-space tabs since otherwise you would end up with something like this:
var jedis = ['luke', 'obi-wan', 'yoda'], sith = ['vader', 'maul', 'sidious'], force = 'with you', x, y, width, height;
Argument 2: Single var
statements are lighter
Well, yes, technically that’s right. Only having a single var
statement for all of your variables means you will be saving about 32 bits per variable (4 characters at 8 bits per). But, even if you have 1,000 variables in your code, that only amounts to a savings of roughly 32kb. That’s not nothing, granted, but in most cases it would end up saving you only a few kb. In 99% of cases, this would be a micro-optimization and your time would be much better suited towards other, more rewarding optimizations such as gzipping, compressing images, reducing the total number of requests, etc…
Besides, minification will automatically convert multiple var
statements into one single var
anyways. And you’re all already minifying your code, right? This truly was the best argument single-var statements had going for it for a long time, but now that we have preprocessors such as Grunt or Gulp, minification is painless and should 100% be part of your workflow.
Argument 3: Comments
Providing insightful, thorough comments in your code is an invaluable practice that should never be overlooked, both for other coworkers as well as future-you. When you have all of your variables declared with a single-line var
statement though, comments become tedious, if not just impossible.
For instance:
var jedi, sith, x, y; // How do I even comment on each of these?!
This isn’t an issue though when you split the variables onto multiple lines.
var jedis, // Array<String> sith, // Array<String> force = 'with you', // String x, // Float y, // Float width, // Float height; // Float
Nor is it an issue when declaring each var
separately.
var jedis; // Array<String> var sith; // Array<String> var force = 'with you'; // String var x; // Float var y; // Float var width; // Float var height; // Float
Argument 4: Commenting-out
Occasionally, as programs evolve, we realize that we don’t need all of the variables we initially declared. We don’t necessarily want to delete them altogether though. We just want them temporarily commented out. You know, just in case.
For the single-line variety, I’m sure I don’t need to explain why it would be annoying to comment out a single variable.
var jedis, //sith, x, y;
var jedis, /*sith,*/ x, y;
For the multiline-single-var variety though, it’s a little less problematic:
var jedis = ['luke', 'obi-wan', 'yoda'], // sith = ['asparagus', 'cauliflower', 'celery'], force = 'with you', x, y, width, height;
However, it’s still an issue if we want to comment out either the first or last variables.
//var jedis = ['luke', 'obi-wan', 'yoda'], sith = ['asparagus', 'cauliflower', 'celery'], force = 'with you', x, y, width, height; // Error! Uncaught ReferenceError: x is not defined
Without the var
statement it looks like we’re trying to reference variables instead of declaring them. But since x, y, width,
and height
were being declared without setting them to a particular value, the JavaScript interpreter fails and throws an error saying you’re trying to reference something that doesn’t exist.
What if we fix that by making sure that all variables are declared right away. (Remember, even null
is considered as having a value).
//var jedis = ['luke', 'obi-wan', 'yoda'], sith = ['vader', 'maul', 'sidious'], force = 'with you', x = null, y = null, width = null, height = null; // Yay! No errors!
Great, everything works fine. Except, we have a new issue now that might not make itself apparent until much later on. Since we didn’t specifically state that we’re declaring variables in our current scope, these variables (vegetables, state, x, y, width, height
) now all belong to the global scope. Meaning if these variables were actually supposed to be parameters to a Class-type object, and you wanted to instantiate multiple instances of that object, than all of these variables would be colliding/overwriting each other.
Depending on the environment, we also have problems if we try and comment out the final variable.
var jedis = ['luke', 'obi-wan', 'yoda'], sith = ['vader', 'maul', 'sidious'], force = 'with you', x, y, width, // height;
Not only are we now missing the statement-terminating semicolon, but we also have this hanging comma from the width
variable. Sometimes that’ll cause a problem; sometimes it won’t. But no matter what it’ll look like a really junior mistake.
Argument 5: Reorganization
Changing around the order of your variables won’t actually change how your program functions, but it can often be beneficial for readability sake if you want to keep certain related variables grouped together. For instance, let’s say our example variable list started off looking like this:
var x, jedis = ['luke', 'obi-wan', 'yoda'], sith = ['vader', 'maul', 'sidious'], y, height, force = 'with you', width;
We would probably want to make sure that x
, y
, width
and height
were declared close together since they all have to do with a related aspect of our object (the geometry). Also, we might want to make sure that variables that have an initial value are declared before those that are left null
. Having to reorganize using the single-var method is annoying. Our first pass looks like this:
jedis = ['luke', 'obi-wan', 'yoda'], sith = ['vader', 'maul', 'sidious'], force = 'with you', var x, y, width; height,
Having to do the same reorganization with the split-var method though is effortless.
Conclusion
Here are the pros and cons for using each method.
Single-var, single-line
- It (subjectively) looks better.
- It weighs less in terms of byte-size. (Only if minification is not part of your workflow).
- It’s impossible to write comments for each variable.
- It’s tedious to comment-out a variable since you have to use multiline commenting.
- It can easily create very long, ugly lines of code that either require wrapping to be turned on, or a horizontal scrollbar to appear.
Single-var, multiline
- It (subjectively) looks better, but only if you have 4-space tabs set in your editor.
- It weighs less in terms of byte-size. (Only if minification is not part of your workflow).
- Writing comments for a variable is effortless.
- Commenting-out a variable is problematic when you need to remove either the first or last variable.
- Reorganizing the order of the variables is problematic in that you need to make sure to also move the
var
statement and the trailing semicolon.
Split-var
- More consistent visually when being edited by more than one developer since the number of spaces used for tabs varies from dev to dev. Things will always line up the way they should.
- Writing comments for a variable is effortless.
- Code maintenance is far easier since commenting-out any variable (first, last, anywhere in between) causes no issues.
- Code maintenance is easier since you can move around the order in which the variables are declared effortlessly.
- Heavier, but only if you’re not minifying your code.
- Looks more junior. (Subjective).
I used to be a dedicated, card-carrying member of the single-var camp. I’ve since reformed my evil ways and realized that split-var is the way to go. Minification is already part of my default workflow (and it should be part of yours too!) and it’s completely effortless given it’s done automatically via a Grunt plugin, so right off the bat we can eliminate the “it’s lighter” argument. But, even if you aren’t minifying your code, I’d argue that the benefits to code maintenance and programmer sanity far outweigh any minor optimizations you might get from saving a measly 32 bits per variable.
To be honest, I’ve actually got my feet in both camps. I do sometimes write multiple variables with a single var
statement, but only when the usage of the variables are so tightly coupled that they would:
- always share the same comment;
- always be commented-out in unison;
- always be moved to another location in unison.
For instance, in the example list of variables I’ve been using throughout this article, I believe this to be the best, most efficient, most programmer-friendly way to write them:
var jedis = ['luke', 'obi-wan', 'yoda']; var sith = ['vader', 'maul', 'sidious']; var force = 'with you'; var x, y; // Tightly coupled. Declare together. var width, height; // Tightly coupled. Declare together.
You missed the keypress argument: not only does the single-var approach save you (meaningless) bytes, it also saves you keystrokes, at least for each subsequent var:
Adding one var to a single-var/multi-line (“foo = bar,”):
*tab*name = value, // two keystrokes (tab and “,”)
Adding one var to a split-var (“var foo = bar;”):
var name = value; // five keystrokes, four from “var “, one from “;”
That’s three whole extra keystrokes per variable! If you write enough (hundreds of thousands? millions?) variables, that will give you carpal tunnel 😉
In all seriousness, great article. Thanks for breaking down the (objective) reasoning for choosing the split-line approach.
In Javascript your conclusions are correct, but what about if we consider language design more generally.
In an indented language (with no need for commas or semicolons) then we can do single line like
var x = 12
or multiline like
var
x = 12
y = 10
width
height
Now there are no problems with removing the first line or the last variable or rearranging. Both of your cons against single-var multiline go away.
var is pretty short, so it’s no big pain to write it on each line, but if you have any useful modifiers on a variable then it’s more of a pain to write each line.
For instance, the export modifier is used to make a defined variable accessible outside of it’s module, then declarations would look like this:
export const double = (x) -> x * 2
export const triple = (x) -> x * 3
This gets tiring, but is pretty concise when doing it multiline:
export const
double = (x) -> x * 2
triple = (x) -> x * 3
Previous message removed indentations from my examples.
Test
Indented
Indented
Indeed, if you’re programming in a language that allows you to get away with not adding the commas or the semicolon, then yeah, go for it. However, this article was specific to JavaScript variable declarations in particular.
The biggest danger with the single-var, multiline method for JavaScript is that if you forget to put a comma at the end of one of the variables, JS will assume a semicolon, and so all the following variables will be treated as being in the global scope. If you’re careful, then it’s not a big deal. But everyone messes up once in a while and it’s one of the worst kinds of errors because it’s not technically an error. You won’t be warned at any point that your variable is polluting the global scope and it can take a very long time to try and debug why certain variables are getting overwritten all because you forgot to put a comma somewhere.
I’m fully in the split-var camp. I don’t care that typing var on each line is 3 more keys. I care that every time I add or remove a variable I have don’t have to look at the other lines to see if I need to add a var or add a comma or remove a comma etc. Everytime I miss one it adds another iteration of either “why isn’t my code minifying? Oh because I forgot this comma” or “why isn’t the code running correctly? Oh because I forgot that comma”. I find when I have split-var style I almost never run into those issues and that totally surpasses saving a few keystrokes.
Same with trailing commas. The only 2 arguments for leaving out trailing commas are IE8 and 1 extra character. Well miinfiiers handle IE8 if I still cared so that reasons disappears. The extra character as the same issue as above where every time I forget a comma, like when adding a property to the end of an object or another element to an array and forgetting to put a comma on a previous line I want to punch an angel. I shouldn’t have to care about the previous line. It has nothing to do with the line I’m adding. Always having the trailing comma means I don’t have to care which means less work, less to think about, less getting compile or runtime errors and slowing me down.